1. 前言
基本思想是:将整数按位数切割成不同的数字,然后按每个位数分别比较。具体做法是:将所有带比较数值统一为同样的数位长度,数位较短的树前面补零。然后,从最低位开始,依次进行一次排序。
这样从最低位排序一直到最高位排序完成以后,数列就变成一个有序序列
2. 实现
通过基数排序对数组{50,3,542,748,14,214,154,63,616},它的示意图如下:
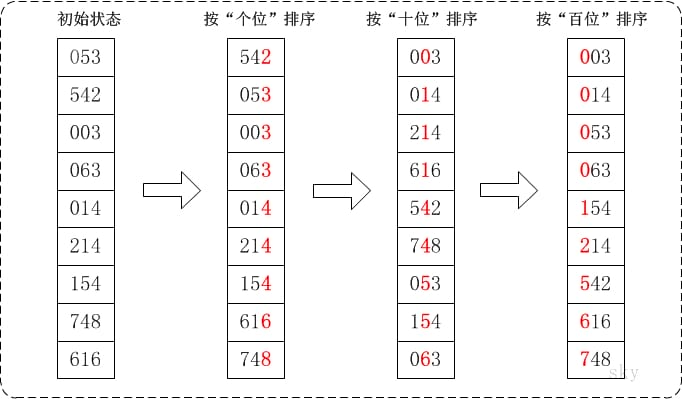
在上图中,首先将所有带比较数值统一为同样长度位数,接着从最低位开始,一次进行排序。
按照个位数进行排序
按照十位数进行排序
按照百位数进行排序
下面简单介绍一下对数组{53, 3, 542, 748, 14, 214, 154, 63, 616}按个位数进行排序的流程:
3. 代码实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81
| package org.example.sort;
public class RadixSort {
private static int getMax(int[] a){ int max = a[0]; for (int i = 1; i < a.length; i++) { if (a[i] > max){ max = a[i]; } } return max; }
private static void countSort(int[] a, int exp){ int[] output = new int[a.length]; int[] buckets = new int[10];
for (int i = 0; i < a.length; i++) { buckets[(a[i]/exp) % 10]++; }
for (int i = 1; i < 10; i++) { buckets[i] += buckets[i - 1]; }
for (int i = a.length - 1; i >= 0; i--) { output[buckets[(a[i] /exp)%10] - 1] = a[i]; buckets[(a[i] /exp)%10] --; } for (int i = 0; i < a.length; i++) { a[i] = output[i]; } output = null; buckets = null; }
public static void radixSort(int[] a){ int exp ; int max = getMax(a); System.out.println(); for(exp = 1; max / exp > 0; exp *= 10){ countSort(a, exp); } }
public static void main(String[] args) { int[] a = {53, 3, 542, 748, 14, 214, 154, 63, 616}; radixSort(a); for (int j : a) { System.out.printf("%d ", j); }
} }
|